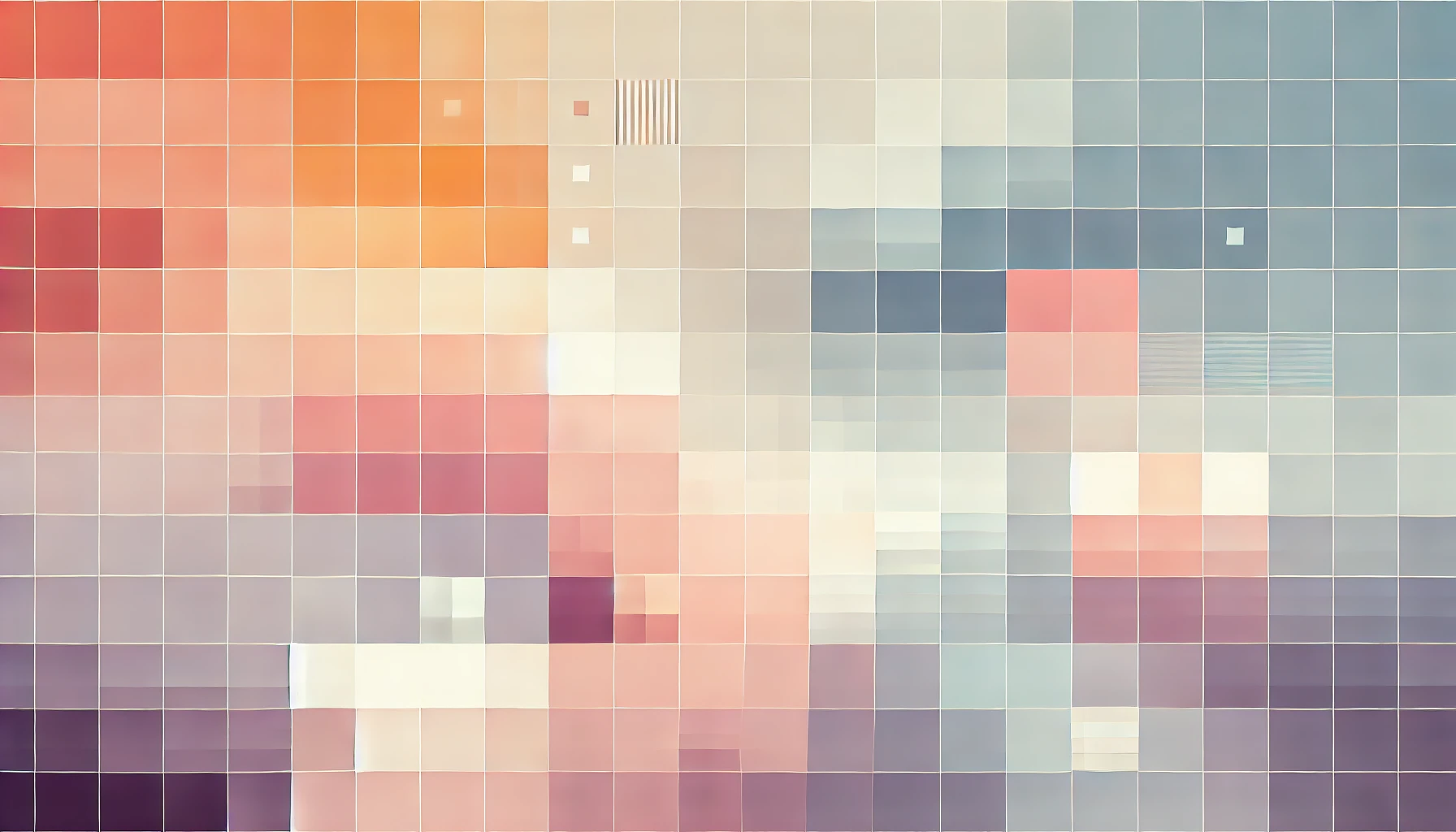
How To Create A Gallery Custom Post Type On Wordpress
When I create custom WordPress theme for clients, I try to avoid needless, un-white-labelled and bloated plugins. So when it comes to creating a simple gallery page, I prefer to design my own post loop in a grid and set a custom post type which makes it easy for the end-client to manage Gallery posts and images. (For those opting for a completely modular approach, you should create a custom plugin to handle custom post types).
In this tutorial I will walk you through the absolute basics of creating a Gallery custom post type on WordPress. I will not focus on creating the post-loop page too much as this will vary depending on how you want your posts to be displayed.
How to create a Gallery custom post type on WordPress
After following this tutorial you will have:
- A custom 'Gallery' post type that appears separately under the 'Posts' tab in your WordPress dashboard.
- A backend post list which displays the thumbnail used in your 'Gallery' post.
- A customised 'Gallery' post editor page to suit the needs of a Gallery custom post type.
Step 1: First of all, we need to create a custom post type and register it to our theme. You should be using a Child Theme for obvious reasons - so go ahead and open your functions.php file.
We are going to add a function to register the Gallery custom post type. The basic code we need is below, but you can get a better idea of what you can customise from the WordPress Codex.
add_action( 'init', 'add_gallery_post_type' );
function add_gallery_post_type() {
register_post_type(
'zm_gallery',
array(
'labels' => array(
'name' => __( 'Gallery' ),
'singular_name' => __( 'Gallery' ),
'all_items' => __( 'All Images')
),
'public' => true,
'has_archive' => false,
'exclude_from_search' => true,
'rewrite' => array(
'slug' => 'gallery-item'),
'supports' => array('title', 'thumbnail' ),
'menu_position' => 4,
'show_in_admin_bar' => false,
'show_in_nav_menus' => false,
'publicly_queryable' => false,
'query_var' => false
)
);
}
The code above registers a custom post type with the id `zm_gallery`` with the name 'Gallery'. It also prevents WordPress from creating a post page for the Gallery items, since they're going to be displayed on a custom page and not have their own page as standard blog posts do.
If you refresh your WordPress dashboard, you will see a new tab under 'Posts' called Gallery. From here, you can add a new Gallery custom post type post. Give it a go and you will notice there is only the option to enter a title and set a featured image. This is thanks to the line *'supports' => array( 'title', 'thumbnail' )* in the code above.
You will also notice there is no way to 'Preview' or 'View' any Gallery custom post type you publish to your website. This is as a result of the last four arguments in the code above - and is suitable for a website where we want to online display the images on a page and disallow access to viewing a page with just the image by the backend user.
**Step 2:** Now, to improve usability of the backend of the website, we will want to add the thumbnail to the admin list of Gallery posts in the WordPress Dashboard. If you now view the backend 'Gallery' tab you will see that posts are listed as 'Title' and 'Date published'. We will now add a column called 'Image' so whoever is using the backend doesn't have to open the post editor to view the image.
To do this, add the following code to your *functions.php* file:
```php
function zm_get_backend_preview_thumb($post_ID) {
$post_thumbnail_id = get_post_thumbnail_id($post_ID);
if ($post_thumbnail_id) {
$post_thumbnail_img = wp_get_attachment_image_src($post_thumbnail_id, 'thumbnail');
return $post_thumbnail_img[0];
}
}
function zm_preview_thumb_column_head($defaults) {
$defaults['featured_image'] = 'Image';
return $defaults;
}
add_filter('manage_posts_columns', 'zm_preview_thumb_column_head');
function zm_preview_thumb_column($column_name, $post_ID) {
if ($column_name == 'featured_image') {
$post_featured_image = zm_get_backend_preview_thumb($post_ID);
if ($post_featured_image) {
echo '';
}
}
}
add_action('manage_posts_custom_column', 'zm_preview_thumb_column', 10, 2);
The first function is used to get the thumbnail for a post. The second function declares the configuration for the column header that will display the Featured Image. The third function is added using a hook and creates the column and loads the thumbnails for each post when any Posts or Page list is called in the WordPress backend.
Step 3: Now we need to clean-up some display configurations in the backend. Load the 'Gallery' tab and you will now see an Image column with the thumbnail for each post - if one has been set via the Featured Image.
Load up the Posts list, and any other Custom Post type your theme may have and you will see the same thing. For my project, I did not necessarily need to display the thumbnail for standard posts. So, upon loading the list, click Screen Options in the top right corner of the page and deselect Image from the drop down menu that appears.
Step 4: Finally, I will show you the basic code for a WP Query that will call all posts under the Gallery custom post type. You can use the WordPress Codex to customise the Query and the way posts are displayed on the page.
Here is the basic code to query for Gallery post types and display the thumbnail:
<?php
$query = new WP_Query(
array( 'post-type' => 'zm_gallery'
);
if ( $query-> have_posts() ) :
while ( $query->have_posts() ) :
$query->the_post();
the_post_thumbnail('thumbnail');
endwhile;
endif;
?>
You can add divs and custom styling to display the thumbnails to your preference.
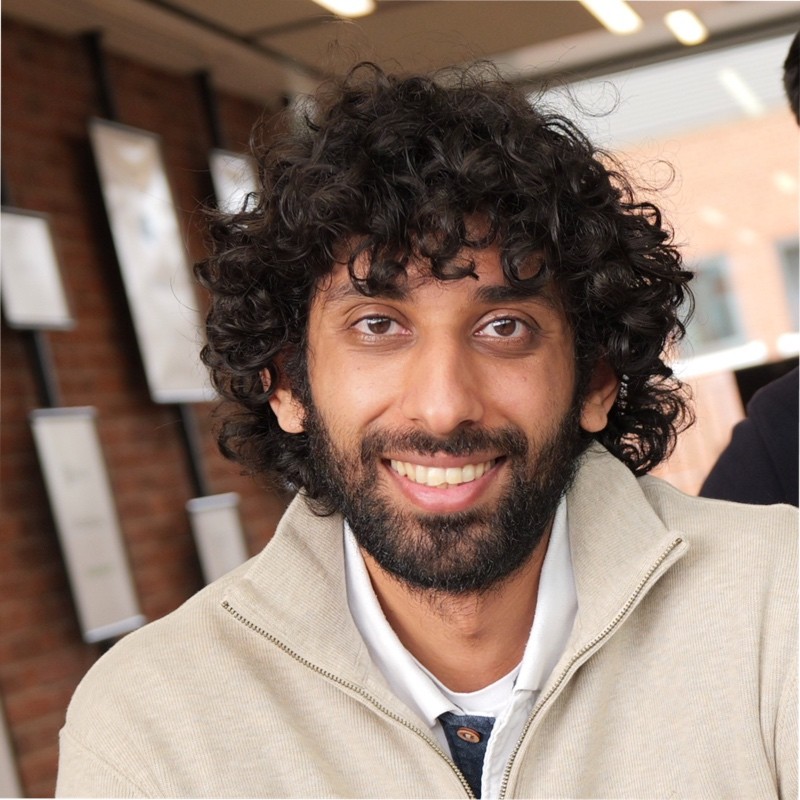
Thanks for reading!
My name is Zahid Mahmood, and I'm one of the founders of Anterior. I started this technology blog when I was in high school and grew it to over 100,000 readers before becoming occupied with other projects. I've recently started writing again and will be posting more frequently.