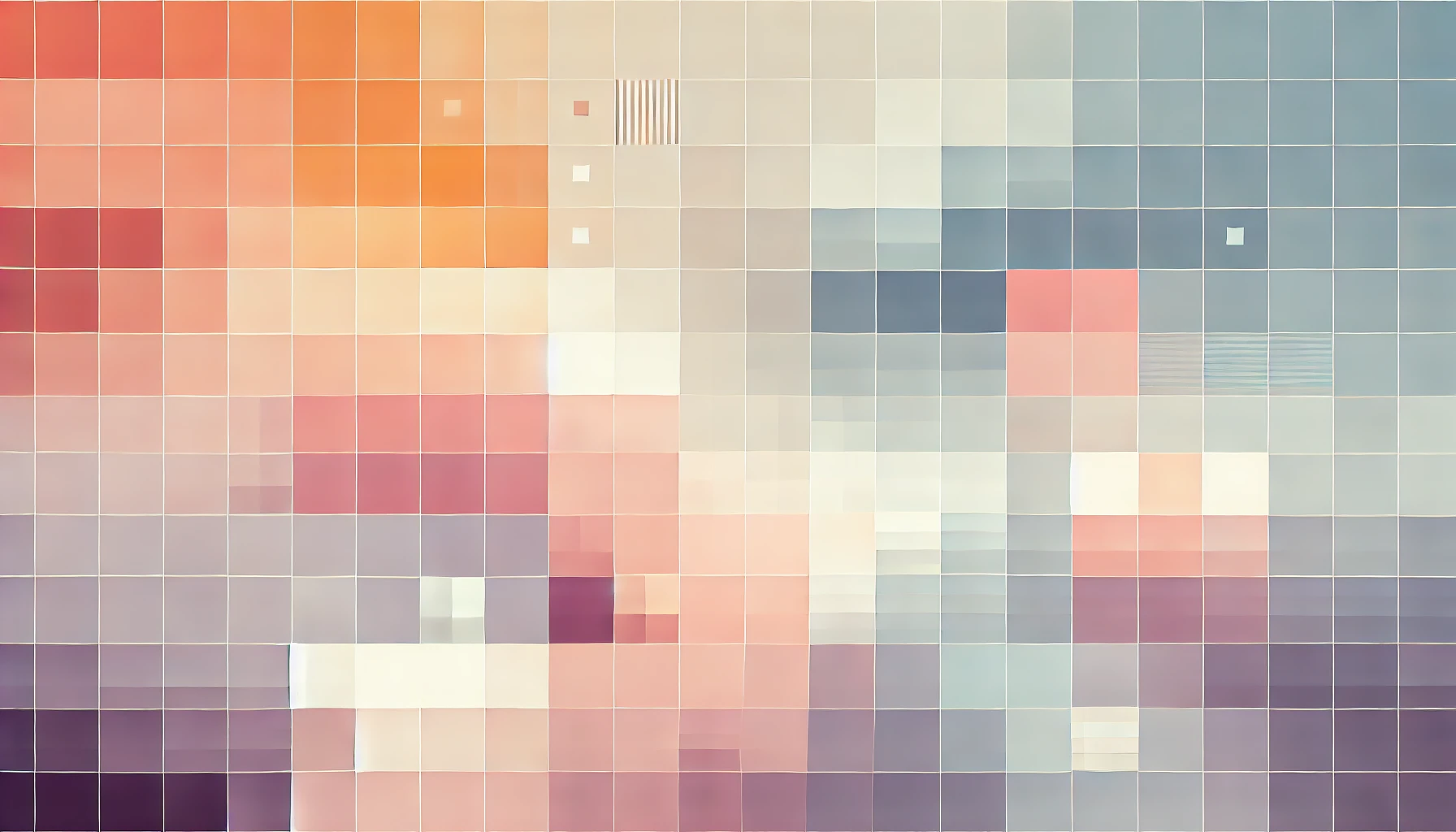
Tailwind CSS v2.0 With Next.js
With the epic release of Tailwind CSS v2.0, here's a guide on how you can add it to a new or existing Next.js project.
1. Create a new Next.js project
Run the following command to create a new Next.js project. This step should not be new to you if you have previously used Next.js:
npx create-next-app
2. Installing TailwindCSS
postcss
v8 or newer is required for Tailwind v2.0. You'll also need a package called autoprefixer
which handles adding vendor-specific prefixes to the compiled CSS (eg. -webkit
).
Install these packages by running the following command in the root of your Next.js project created in Step 1:
npm install tailwindcss postcss autoprefixer --save
3. Configuring PostCSS
The next step is to configure postcss
to work with tailwindcss
and autoprefixer
. Start by creating a file called postcss.config.js
in the root of your Next.js project. This can be done from the command line using the command:
touch postcss.config.js
Then, add the following code to the postcss.config.js
file:
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {}
}
}
4. Add Tailwind styles to the project
Now that postcss
is configured to work with tailwindcss
, we want to add Tailwind to the project. Create a file called tailwind.css
in the /styles
directory of your Next.js project and add the following code:
// styles/tailwind.css
@tailwind base;
@tailwind components;
@tailwind utilities;
The @tailwind
directive is used by postcss
to inject the Tailwind base CSS, components CSS and utilities CSS in to the compiled stylesheet.
Using TailwindCSS in your Next.js project
Start by importing the TailwindCSS styles globally. To do this, open the pages/_app.js
file and add the following line of code at the top of the file:
// add this line
import "../styles/tailwind.css";
...
// the code below should already be in the _app.js file
function App({ Component, pageProps }) {
return ...
}
If you were already running the project with npm run dev
, you will need to stop the development server and restart it to ensure the changes take effect.
Testing TailwindCSS is installed correctly
You can test if you have followed the steps above correctly by replacing the contents of pages/index.js
with the following code:
export default function Home() {
return (
<h1 className="text-3xl text-indigo-400 bg-gray-300 p-4 text-center">
Purple title with gray background
<h1>
);
}
This should then display a purple title and gray background, styled by Tailwind at localhost:3000
!
Bonus: Customising TailwindCSS
One of the powerful features of Tailwind is how it can be customised. Although the default configuration, palettes and styles work great for beginners, at some poibt you'll want to customise them for your project.
To do this, first create a Tailwind config file by running the following command in the root of your Next.js project:
npx tailwindcss init
This will create a tailwind.config.js
file in the root directory with the following content:
module.exports = {
purge: [],
darkMode: false,
theme: {
extend: {},
},
variants: {},
plugins: [],
}
You can now use the official Tailwind customisation docs for guidance on how to customise Tailwind for your project.
If you haven't already - check out the epic hype video for TailwindCSS v2.0 here:
https://www.youtube.com/watch?v=3u_vIdnJYLc
Happy coding!
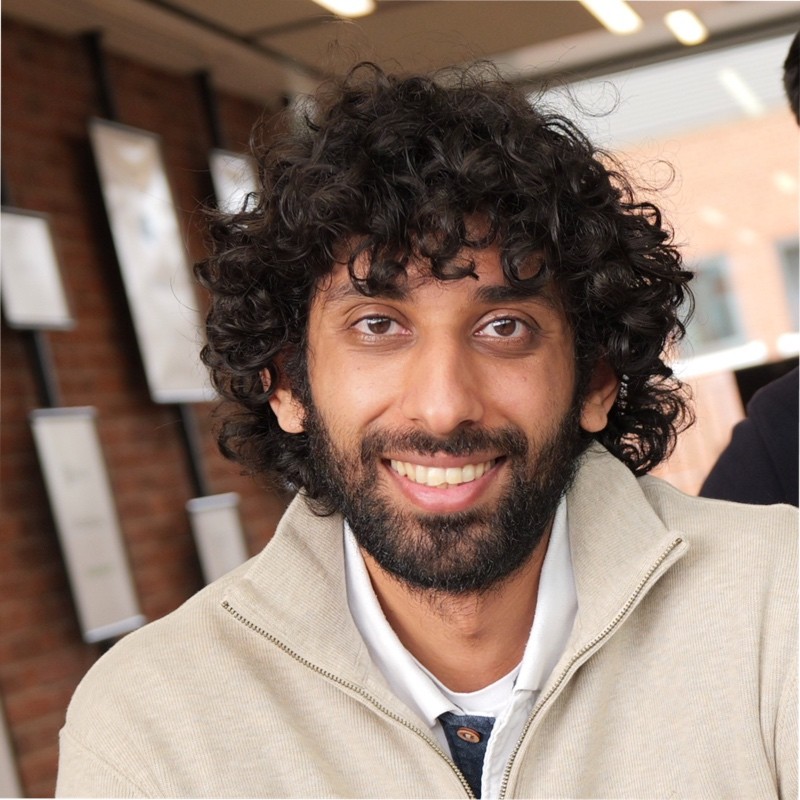
Thanks for reading!
My name is Zahid Mahmood, and I'm one of the founders of Anterior. I started this technology blog when I was in high school and grew it to over 100,000 readers before becoming occupied with other projects. I've recently started writing again and will be posting more frequently.