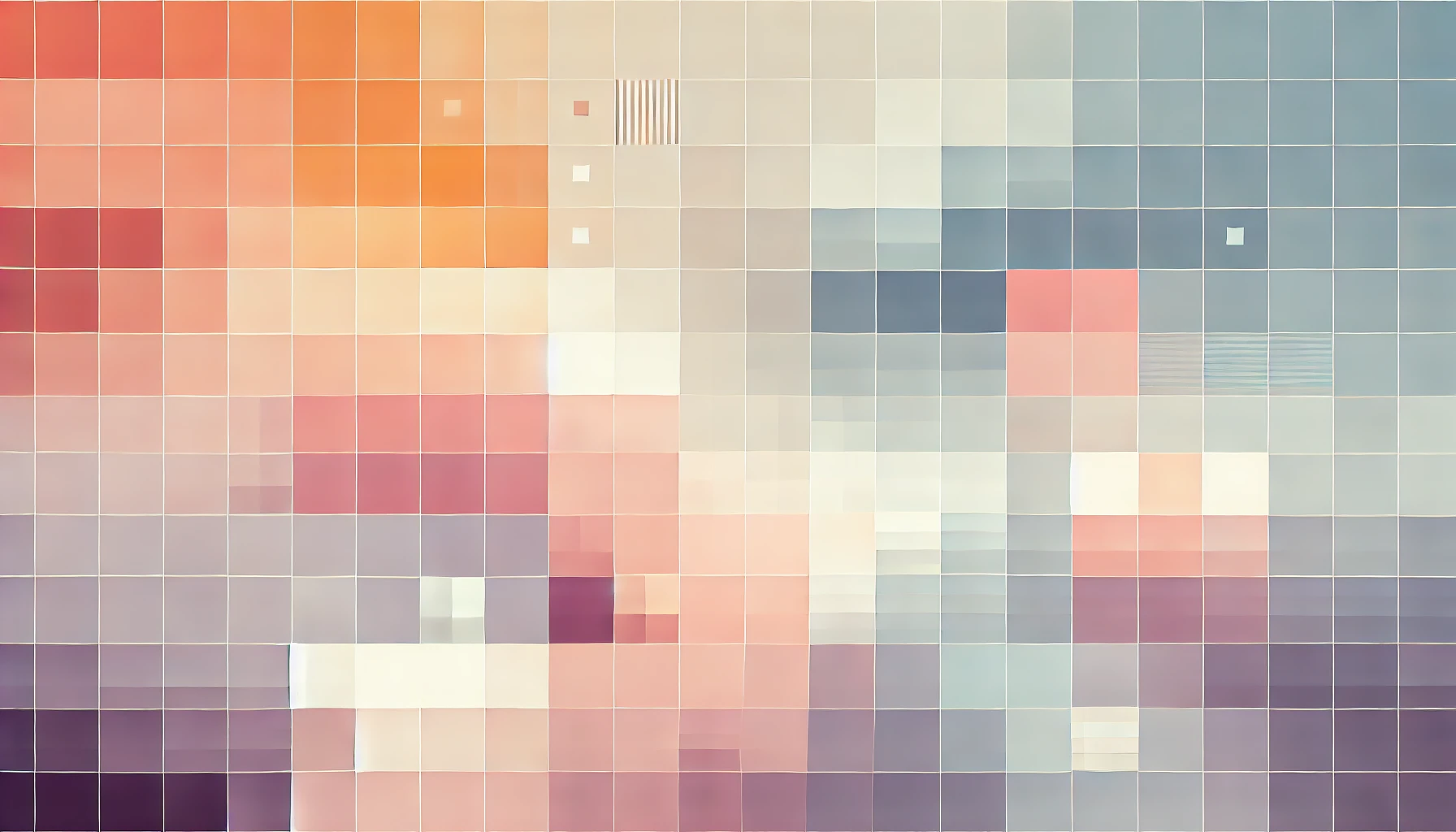
Ionic Automated Build Script For iOS & Android (macOS)
The Ionic framework does a great job of making the build process from JavaScript to a bundled Android APK or Apple XCode Project really simple. In most cases, you only need to run the command line instruction ionic build [platform]
from within your project. However the process to build for both Android and iOS, and then sign the APK for the Google Play Store can be cumbersome.
This post will show you how you can write your own custom build script for macOS which will build your application for both iOS and Android, and also sign the Android APK ready to be uploaded to the Play Store. iOS apps will still need to be opened in XCode, archived and then uploaded to iTunes Connect but at least you can run a single command and grab yourself a coffee!
I will update this post when I find out how to completely automate the iOS/XCode process. If you have any contributions please tweet me.
Writing an automated build script for Ionic
This section will break down what each line does. If you just want the code, scroll to the end!
Step 1: Create a file called build
(with no extension) and save it to the root of your Ionic source-code. On the first line of the file add:
#!/bin/bash
Any lines which start with echo
are just to print information to the screen, similar to console.log()
in JavaScript.
Step 2: Let's start with iOS first.
echo iOS build command started...
ionic cordova build ios --prod
echo iOS build command finished.
echo XCode project file can be found in platforms/ios/APP_NAME.xcodeproj
This block of code writes a message to the console before running the standard ionic build
command. Once completed there is a confirmation message. You should replace 'APP_NAME' with something more appropriate.
Step 3: Next, we run the standard ionic build
command for Android:
echo Android build command started...
ionic cordova build android --prod --release
echo Android build command finished.
Step 4: Next, we use our keystore
to sign the Android APK:
echo Jarsigner command started...
jarsigner -verbose -sigalg SHA1withRSA -digestalg SHA1 -keystore APP_NAME.keystore platforms/android/app/build/outputs/apk/release/app-release-unsigned.apk APP_NAME -storepass KEYSTORE_PASSWORD
echo Jarsigner command finished.
Remember, to replace 'APP_NAME' and 'KEYSTORE_PASSWORD' with something more appropriate.
Step 5: Next, as according to the Ionic documentation is the zipalign
command:
echo Zipalign command started...
~/Library/Android/sdk/build-tools/27.0.3/zipalign -v 4 platforms/android/app/build/outputs/apk/release/app-release-unsigned.apk platforms/android/app/build/outputs/apk/release/APP_NAME.apk
echo Zipalign command finished.
You may need to change the version of your Android SDK build tools. My machine was using Zipalign v27.0.3
.
Step 6: And finally we add one more section for the apksigner
command:
echo Apksigner command started...
~/Library/Android/sdk/build-tools/27.0.3/apksigner verify platforms/android/app/build/outputs/apk/release/APP_NAME.apk
echo Apksigner command finished.
The full build
file show now look something like this:
#!/bin/bash
echo iOS build command started...
ionic cordova build ios --prod
echo iOS build command finished.
echo XCode project file can be found in platforms/ios/APP_NAME.xcodeproj
echo Android build command started...
ionic cordova build android --prod --release
echo Android build command finished.
echo Jarsigner command started...
jarsigner -verbose -sigalg SHA1withRSA -digestalg SHA1 -keystore APP_NAME.keystore platforms/android/app/build/outputs/apk/release/app-release-unsigned.apk APP_NAME -storepass KEYSTORE_PASSWORD
echo Jarsigner command finished.
echo Zipalign command started...
~/Library/Android/sdk/build-tools/27.0.3/zipalign -v 4 platforms/android/app/build/outputs/apk/release/app-release-unsigned.apk platforms/android/app/build/outputs/apk/release/APP_NAME.apk
echo Zipalign command finished.
echo Apksigner command started...
~/Library/Android/sdk/build-tools/27.0.3/apksigner verify platforms/android/app/build/outputs/apk/release/APP_NAME.apk
echo Apksigner command finished.
To run the build process, open Terminal and navigate to the root of your Ionic project, which now also contains the build
file defined above. Then, instead of running ionic build [platform]
you can run the command ./build
.
You'll then need to open the xcodeproj
file for publishing to the App Store and upload the signed APK to the Play Store.
This article was inspired by the cumbersome build and publish process I experienced whilst working with Halal Joints - Halal Food Finder App built with Ionic.
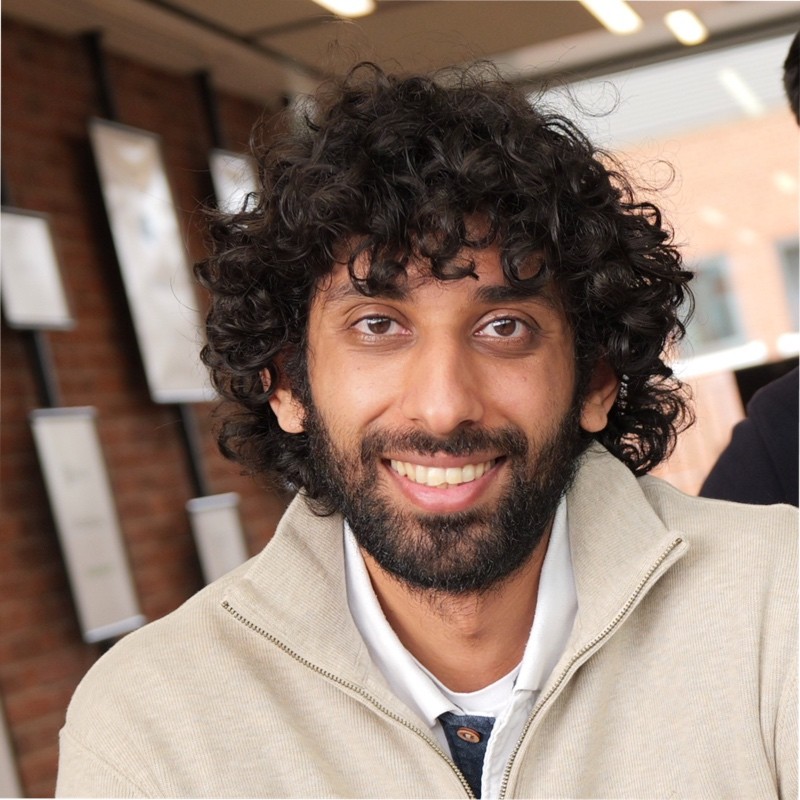
Thanks for reading!
My name is Zahid Mahmood, and I'm one of the founders of Anterior. I started this technology blog when I was in high school and grew it to over 100,000 readers before becoming occupied with other projects. I've recently started writing again and will be posting more frequently.